Mobile App Development with React Native & Firebase
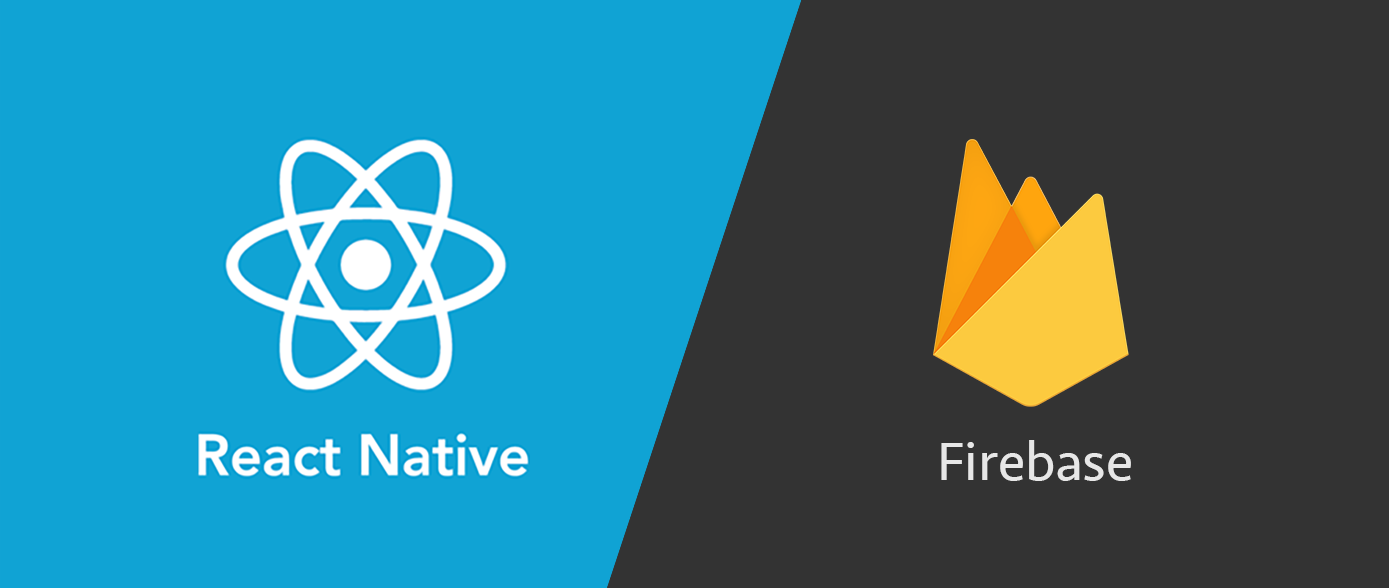
Facilitated by Misha Khlioustov & Bobby Henderson
React Native and Firebase are a powerful combination of technologies used for building cross platform and easy to manage mobile applications. Below are helpful resources for both React Native and Firebase.
Meetings
This meeting was conducted face to face, prior to COVID-19. Therefore, no recordings are available. However, we do have a step by step guide.
Resources & Links
Below are links with more information on various React JS/Native concepts. Some of which we've covered in our meetings.
Components
Styling Components with StyleSheet
Alternative Component Libraries
Setting up navigation from scratch
React Native Layout (Flexbox)
Step by Step Guides
Getting Started with Expo Cli
One of the easiest ways to get started with React Native is by using Expo to quickly start and run your Native projects. Below are instructions for installing the Expo CLI along with setting up your app.
Requirements
- node.js installed https://nodejs.org/en/download/
- visual studio code or an alternative IDE https://code.visualstudio.com/download
- expo client app installed on your iOS / Android phone
Install Expo: ('$' means it's a command)
- Open cmd (command prompt) and run the following commands:
- $ npm install -g expo-cli
- $ expo init RecipeApp
- After this command, you should select the following option: > tabs . . . . . . . navigation
- After that, it will prompt you to name your app again.
- $ cd RecipeApp
- $ npm start
Development Server
- If at home, use LAN if you're connected to the same network. This is usually faster. Scan the QR code.
- If at Broward, use Tunnel. Scan the QR code.
Alternatives to Expo:
- use a web alternative https://snack.expo.io/
Links:
Creating a Database with Firebase
- Firebase allows you to create a database on their console. To do so, go to firebase.google.com, and create a project. Go to the Database tab, and select "Realtime Database". You may go into Test mode so it's easier for us to manipulate information.
- You can create your database according to how you think it will best be defined. For this project, I'm categorizing our meals by "Breakfast", "Lunch", and "Dinner". So I'll start by creating a "Lunch" category and filling in records.
- When you're in the database, you'll see a plus sign near your project name. Click that, and type in "Lunch" where it says "name". Don't give it a value. Click the "add child" in the Lunch table you made. Now we can add records.
- A database record MUST have a unique identifier that separates it from other records. If you think about it, we can have a school with two "John Rojas"'s, but they are two completely different people. And they have different student IDs.
- So when you want to branch out into a new table, you give the table a name but give it a null value (meaning you don't enter anything in the "value" field), and click the plus sign to add more entries. Our first record will have the name "id0001", and I will branch out into a new table.
-
So let's start by making a HOT DOG!
First name is going to be "title" with the value "Hot dog"
Next is "ingredients" with the value "bread, sausage"
Next is "time" with the value "3 minutes" - Now we are at "instructions", which has multiple values. Let's branch that into another table for easy readability. Add the name and value pairs according to however you cook Hot dogs.
- Now we have at least one record in our database. Feel free to create more records and try to follow the consistent structure of "title", "ingredients", "time", and "instructions".


Connecting with React Native
- In your terminal, run the command "npm install firebase"
- Now we need to get all the config code from Firebase. Go into your Firebase settings: Settings > Your Apps > the icon ""
- Now register your app.
- Copy the config code. It looks something like this:
- Now go back to your project folder, and create a new component under your screens folder. Call it "LunchScreen.js" or something. Let's start with our imports, and proceed into making our necessary imports. For Firebase, we must make a require statement.
- Start out your component by declaring it as a smart (class) component. "class Whatever extends Component {}". Include the render() and the return() statements as well.
- Above the render() function, we need to declare our initial states.
- Loading is initially set as true because when you first stumble onto a page, it must load and process the components that are about to be rendered. The meals list is set as an empty list. This will change once we form our query.
- Let's start our query function using arrow function notation.
- We need to reference the "Lunch" database and retrieve all the values. The retrieval parameter in this case will be referenced as "snapshot", and the value we really want is snapshot.val(), so we will store that in an Object.
- Then, we must add the unique identifier in the object and translate it into a simple list, which is done in the next line of code.
- At the very end, we change the state of "meals" and "loading" since we now have the list. Loading is set to true because we want it to display the database records instead of the text "Loading".
- Now we have finished defining the query function. But at this point in our component, we have never called it. So we need to call it in the componentWillMount() lifecycle function.
- Now the final step is displaying the information in the return() statement, and then linking this screen to the MainTabNavigator.
- To write actual JavaScript code in the return statement, you need to enclose it in curly braces. We need to write a conditional statement for displaying the database records.
- Here it is in ENGLISH:"If loading is true, then display the text "Loading . . .". Else (if it's not true), display the value of this.state.recipes."
- Here it is in CODE:
- And since {this.state.recipes} is an array, we need to map it out so that we can display each element of the array properly, rather than just spit it all out right then and there.
- As you can see, we are referencing the names of each piece of information that we defined in our database. "meals.title", "meals.ingredients" . . etc . . .
- We also have one piece of information that branches out into another table. We need to map that one out as well.
- I missed this step. Don't forget to put a parent container around all the database information.
- Now we must export the component and import it and use it in the MainTabNavigator.
- For more information on how to connect React Native to Firebase database, refer to the official documentation here https://firebase.google.com/docs/database

